마이크로 소프트에서 나온 엑셀 파일을 직접 프로그램으로 작성해서 만들어 볼 수 있는 것으로...
그런데, 몇 몇 기능을 좀 더 넣고 싶어서 이렇게 시작해 보게 되었다는 것입니다.
해보고 싶은 기능은
- 셀에 색깔 넣고,
- 셀 크기를 글자 크기에 맞게 하고,
- 셀 전체 바운더리 치고
- 한 시트 안에 두개의 테이블 넣고,
- 마지막으로 만들어진 엑셀 파일들을 하나의 엑셀로
만들어 보는 것입니다.
최신 버전의 아파치 POI 를 사용해서 한 번 해보기로 했습니다.
1.아파치 POI 라이브러리 다운 받기
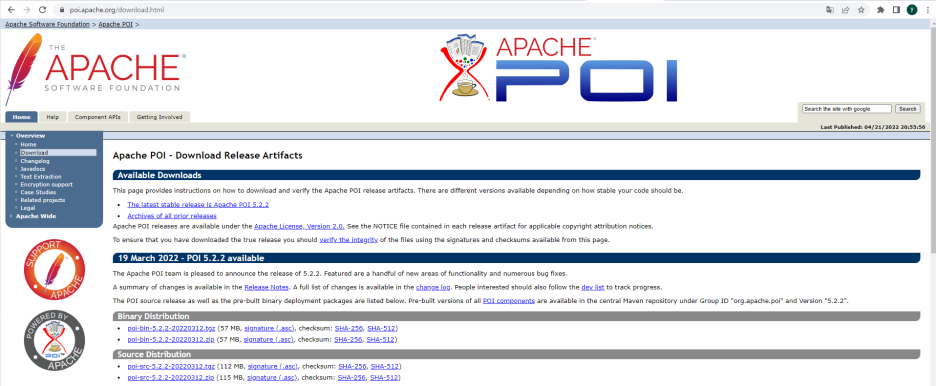
Apache POI - the Java API for Microsoft Documents
<!--+ |breadtrail +--> <!--+ |start Menu, mainarea +--> <!--+ |start Menu +--> <!--+ |end Menu +--> <!--+ |start content +--> Apache POI - the Java API for Microsoft Documents Project News 19 March 2022 - POI 5.2.2 available The Apache POI team is pleased
poi.apache.org
라이브러리 설정을 다음 그림과 같이 해 주었습니다. 이 구성으로 성공 한 것이기 때문에 그대로 하면 될 듯 합니다.
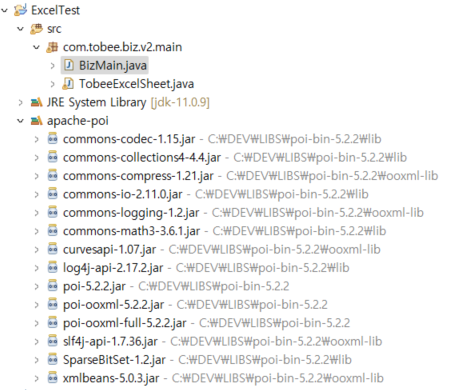
static class MyExcelObj
{
public String getCol1() {
return Col1;
}
public void setCol1(String col1) {
Col1 = col1;
}
public String getCol2() {
return Col2;
}
public void setCol2(String col2) {
Col2 = col2;
}
public String getCol3() {
return Col3;
}
public void setCol3(String col3) {
Col3 = col3;
}
public String getCol4() {
return Col4;
}
public void setCol4(String col4) {
Col4 = col4;
}
public String getCol5() {
return Col5;
}
public void setCol5(String col5) {
Col5 = col5;
}
public String getCol6() {
return Col6;
}
public void setCol6(String col6) {
Col6 = col6;
}
public String getCol7() {
return Col7;
}
public void setCol7(String col7) {
Col7 = col7;
}
private String Col1 = null;
private String Col2 = null;
private String Col3 = null;
private String Col4 = null;
private String Col5 = null;
private String Col6 = null;
private String Col7 = null;
}
위 클래스이 리스트를 받아서 엑셀을 만든다고 한다면 다음 코드가 될 수 있을 것입니다.
public static void exportExcvEOCSToExcel(List<MyExcelObj> myexcelObjList, int nIter)
{
String excelFilePath = "2)myexcel_${count}.xlsx".replace("${count}",String.valueOf(myexcelObjList.size()));
XSSFWorkbook workbook = null;
XSSFSheet sheet = null;
BufferedOutputStream outputStream = null;
final String headerNames[][]
= new String[][]{
{"컬럼1", "컬럼2", "컬럼3", "컬럼4", "컬럼5", "컬럼6", "컬럼7"},
{"Col1", "Col2", "Col3", "Col4", "Col5", "Col6", "Col7"}
};
final int numberOfColumns = headerNames[0].length;
try
{
workbook = new XSSFWorkbook();
sheet = workbook.createSheet(excelFilePath);
Row headerRow = sheet.createRow(0);
String columnName = null;
for (int i = 0; i < numberOfColumns; i++) {
columnName = headerNames[0][i];
Cell headerCell = headerRow.createCell(i);
headerCell.setCellValue(columnName);
columnName = null;
}
} catch (Exception e) {
System.out.println("File IO error:");
e.printStackTrace();
}
String Col1 = null;
String Col2 = null;
String Col3 = null;
String Col4 = null;
String Col5 = null;
String Col6 = null;
String Col7 = null;
MyExcelObj selfObj = null;
try
{
int rowCount = 1;
int cellCount = 0;
for(Iterator<MyExcelObj> checkIter = myexcelObjList.iterator(); checkIter.hasNext();)
{
selfObj = checkIter.next();
Row row = sheet.createRow(rowCount++);
cellCount =0;
Col1 = selfObj.getCol1();
Col2 = selfObj.getCol2();
Col3 = selfObj.getCol3();
Col4 = selfObj.getCol4();
Col5 = selfObj.getCol5();
Col6 = selfObj.getCol6();
Col7 = selfObj.getCol7();
Cell cell1 = row.createCell(cellCount++);
Cell cell2 = row.createCell(cellCount++);
Cell cell3 = row.createCell(cellCount++);
Cell cell4 = row.createCell(cellCount++);
Cell cell5 = row.createCell(cellCount++);
Cell cell6 = row.createCell(cellCount++);
Cell cell7 = row.createCell(cellCount++);
cell1.setCellValue(Col1);
cell2.setCellValue(Col2);
cell3.setCellValue(Col3);
cell4.setCellValue(Col4);
cell5.setCellValue(Col5);
cell6.setCellValue(Col6);
cell7.setCellValue(Col7);
Col1 = null;
Col2 = null;
Col3 = null;
Col4 = null;
Col5 = null;
Col6 = null;
Col7 = null;
}
outputStream = new BufferedOutputStream(new FileOutputStream(excelFilePath));
workbook.write(outputStream);
} catch (IOException e) {
System.out.println("File IO error:");
e.printStackTrace();
}
finally
{
try {
if(workbook != null) workbook.close();
if(outputStream != null) outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
뼈대만 본다면 다음과 같이 워크 북을 선언하고, 행과 열을 만들고 각 열마다 데이터를 집어 넣어 주는 작업을 하게 될 것입니다.
public static void exportExcvEOCSToExcel(List<MyExcelObj> myexcelObjList, int nIter)
{
//저장 할 엑셀 파일명 선언
String excelFilePath = "myexcel_${count}.xlsx".replace("${count}",String.valueOf(myexcelObjList.size()));
XSSFWorkbook workbook = null;
XSSFSheet sheet = null;
BufferedOutputStream outputStream = null;
//헤더를 선언하고
final String headerNames[][]
= new String[][]{
{"컬럼1", "컬럼2", "컬럼3", "컬럼4", "컬럼5", "컬럼6", "컬럼7"},
{"Col1", "Col2", "Col3", "Col4", "Col5", "Col6", "Col7"}
};
final int numberOfColumns = headerNames[0].length;
try
{
workbook = new XSSFWorkbook();
sheet = workbook.createSheet(excelFilePath);
// 열을 하나 생성하고
Row headerRow = sheet.createRow(0);
String columnName = null;
// 각 셀에 헤더 값을 집어 넣는다.
for (int i = 0; i < numberOfColumns; i++) {
columnName = headerNames[0][i];
Cell headerCell = headerRow.createCell(i);
headerCell.setCellValue(columnName);
columnName = null;
}
} catch (Exception e) {
System.out.println("File IO error:");
e.printStackTrace();
}
...
try
{
int rowCount = 1;
int cellCount = 0;
for(Iterator<MyExcelObj> checkIter = myexcelObjList.iterator(); checkIter.hasNext();)
{
selfObj = checkIter.next();
//열을 하나 생성하고,
Row row = sheet.createRow(rowCount++);
cellCount =0;
...
//각 셀을 생성하고,
Cell cell1 = row.createCell(cellCount++);
Cell cell2 = row.createCell(cellCount++);
Cell cell3 = row.createCell(cellCount++);
Cell cell4 = row.createCell(cellCount++);
Cell cell5 = row.createCell(cellCount++);
Cell cell6 = row.createCell(cellCount++);
Cell cell7 = row.createCell(cellCount++);
//각 셀의 값을 저장한다.
cell1.setCellValue(Col1);
cell2.setCellValue(Col2);
cell3.setCellValue(Col3);
cell4.setCellValue(Col4);
cell5.setCellValue(Col5);
cell6.setCellValue(Col6);
cell7.setCellValue(Col7);
...
}
//엑셀 파일 생성
outputStream = new BufferedOutputStream(new FileOutputStream(excelFilePath));
workbook.write(outputStream);
} catch (IOException e) {
System.out.println("File IO error:");
e.printStackTrace();
}
finally
{
try {
if(workbook != null) workbook.close();
if(outputStream != null) outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
위의 코드는 최신 버전에서 API가 변경이 있어 오류가 난다는 것입니다~
POI 사이트의 퀵 가이드를 참고 해보도록 합니다.
https://poi.apache.org/components/spreadsheet/quick-guide.html
Busy Developers' Guide to HSSF and XSSF Features
Busy Developers' Guide to HSSF and XSSF Features Busy Developers' Guide to Features Want to use HSSF and XSSF read and write spreadsheets in a hurry? This guide is for you. If you're after more in-depth coverage of the HSSF and XSSF user-APIs, please consu
poi.apache.org
2. 코드 변경하기
변경 된 코드는 그렇게 많지 않습니다.
public static void exportExcvEOCSToExcel(List<MyExcelObj> myexcelObjList, int nIter)
{
String excelFilePath = "2)myexcel_${count}.xlsx".replace("${count}",String.valueOf(myexcelObjList.size()));
Workbook workbook = null;
Sheet sheet = null;
final String sheetName = "MyFirstSheet";
final String headerNames[][]
= new String[][]{
{"컬럼1", "컬럼2", "컬럼3", "컬럼4", "컬럼5", "컬럼6", "컬럼7"},
{"Col1", "Col2", "Col3", "Col4", "Col5", "Col6", "Col7"}
};
final int numberOfColumns = headerNames[0].length;
try
{
workbook = new XSSFWorkbook();
sheet = workbook.createSheet(sheetName);
...
3. 테스트 해보기
테스트 코드는 다음과 같습니다.
package com.tobee.biz.v2.main;
import java.util.ArrayList;
import java.util.List;
public class BizMain {
public static void main(String[] args)
{
List<TobeeExcelSheet.MyExcelObj> myxcelList = new ArrayList<TobeeExcelSheet.MyExcelObj>(); //selfChkService.retrieveExcvSaftyEdu(cmpCod, dateStart, dateEnd);
TobeeExcelSheet.MyExcelObj obj1 = new TobeeExcelSheet.MyExcelObj();
obj1.setCol1("ID_1");
obj1.setCol2("124");
obj1.setCol3("This is my excel");
obj1.setCol4("This is my excel2");
obj1.setCol5("Helloooo");
obj1.setCol6("True or False");
obj1.setCol7("AAAAA");
TobeeExcelSheet.MyExcelObj obj2 = new TobeeExcelSheet.MyExcelObj();
obj2.setCol1("ID_2");
obj2.setCol2("111");
obj2.setCol3("This is my excel");
obj2.setCol4("This is my excel2");
obj2.setCol5("Helloooo");
obj2.setCol6("True or False");
obj2.setCol7("AAAAA");
TobeeExcelSheet.MyExcelObj obj3 = new TobeeExcelSheet.MyExcelObj();
obj3.setCol1("ID_3");
obj3.setCol2("222");
obj3.setCol3("This is my excel");
obj3.setCol4("This is my excel2");
obj3.setCol5("Helloooo");
obj3.setCol6("True or False");
obj3.setCol7("AAAAA");
TobeeExcelSheet.MyExcelObj obj4 = new TobeeExcelSheet.MyExcelObj();
obj4.setCol1("ID_4");
obj4.setCol2("333");
obj4.setCol3("This is my excel");
obj4.setCol4("This is my excel2");
obj4.setCol5("Helloooo");
obj4.setCol6("True or False");
obj4.setCol7("AAAAA");
myxcelList.add(obj1);
myxcelList.add(obj2);
myxcelList.add(obj3);
myxcelList.add(obj4);
try
{
TobeeExcelSheet.exportMyDataToExcel(myxcelList);
}
catch(Exception e)
{
}
}
}
결과는 다음과 같이 아름다운(?) 엑셀 파일입니다...
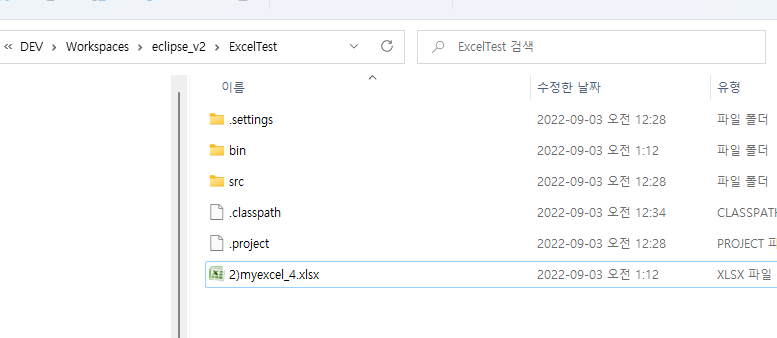
결과 입니다..
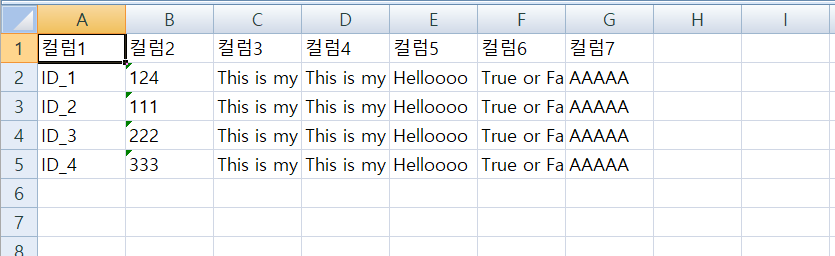
4. 스타일 적용하기
열과 행의 스타일을 적용하는 방법입니다. 퀵스타트 가이드에 따라서 진행 해 보았습니다.
우선 다음 메서드를 가지고 옵니다.
/**
* Creates a cell and aligns it a certain way.
*
* @param wb the workbook
* @param row the row to create the cell in
* @param column the column number to create the cell in
* @param halign the horizontal alignment for the cell.
* @param valign the vertical alignment for the cell.
* @param value the value for the cell.
*/
private static void createCell(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
Cell cell = row.createCell(column);
cell.setCellValue(value);
CellStyle cellStyle = wb.createCellStyle();
cellStyle.setAlignment(halign);
cellStyle.setVerticalAlignment(valign);
cell.setCellStyle(cellStyle);
}
열의 스타일 변경
Row headerRow = sheet.createRow(0);
headerRow.setHeightInPoints(30);
셀 스타일 변경
createCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCell(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCell(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCell(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCell(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCell(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCell(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
위의 코드가 우리의 아름다운 엑셀을 어떻게 변경 시켰는 지 알아봅시다.
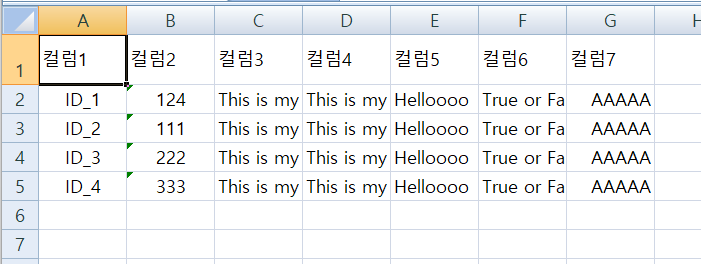
처음 예제와 비교해서 좀 많이 변한 듯 하네요...
createCell2 메서드를 다음과 같이 생성 합니다.
private static void createCell2(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Style the cell with borders all around.
CellStyle style = wb.createCellStyle();
style.setBorderBottom(BorderStyle.THIN);
style.setBottomBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderLeft(BorderStyle.THIN);
style.setLeftBorderColor(IndexedColors.GREEN.getIndex());
style.setBorderRight(BorderStyle.THIN);
style.setRightBorderColor(IndexedColors.BLUE.getIndex());
style.setBorderTop(BorderStyle.MEDIUM_DASHED);
style.setTopBorderColor(IndexedColors.BLACK.getIndex());
cell.setCellStyle(style);
}
테스트 코드은 아래와 같습니다.
createCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCell(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCell(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCell(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCell2(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCell2(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCell2(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
결과는 다음과 같이 나오네요.
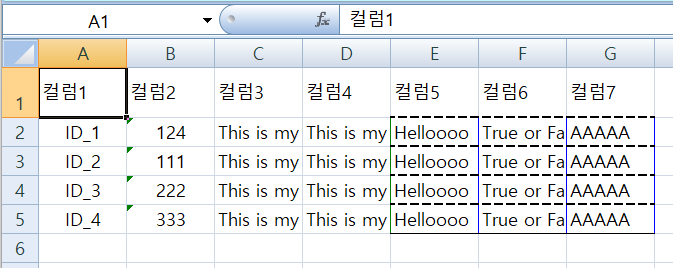
다음은 셀 채우기와 색상에 관련 된 내용으로 createCell3, createCell4 메서드를 만들었습니다.
private static void createCell3(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Aqua background
CellStyle style = wb.createCellStyle();
style.setFillBackgroundColor(IndexedColors.AQUA.getIndex());
style.setFillPattern(FillPatternType.BIG_SPOTS);
cell.setCellStyle(style);
}
private static void createCell4(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Orange "foreground", foreground being the fill foreground not the font color.
CellStyle style = wb.createCellStyle();
style.setFillForegroundColor(IndexedColors.ORANGE.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
cell.setCellStyle(style);
}
테스트 코드 입니다.
createCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCell2(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCell2(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCell3(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCell3(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCell4(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCell4(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
결과를 알아 봅시다.
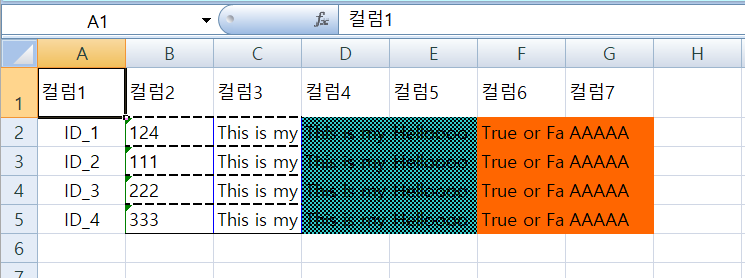
그럼 마지막으로 폰트 작업을 한 번 해 보도록 할까요?
폰트 작업을 하기 전에 내 시스템에 설치 되어 있는 폰트를 먼저 알아보도록 하겠습니다.
스택오버플로우 선생님들이 이미 답변 해 놓으신 내용을 한 번 보고 진행 해 봅시다.
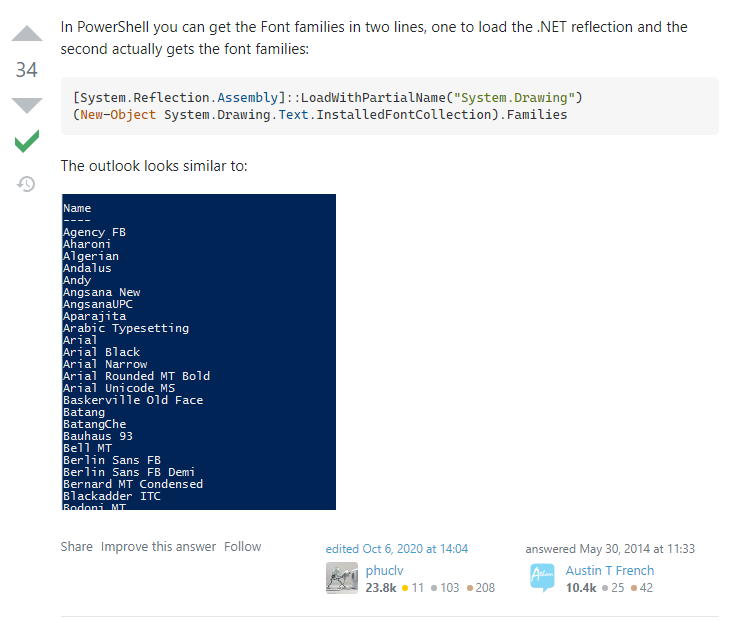
우리의 createCell5 메서드 입니다.
private static void createCell5(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
// Create a new font and alter it.
Font font = wb.createFont();
font.setFontHeightInPoints((short)24);
font.setFontName("HY그래픽M");
font.setItalic(true);
font.setStrikeout(true);
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Orange "foreground", foreground being the fill foreground not the font color.
CellStyle style = wb.createCellStyle();
style.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style.setFont(font);
cell.setCellStyle(style);
}
테스트 코드 입니다.
createCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCell2(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCell2(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCell3(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCell4(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCell5(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCell5(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
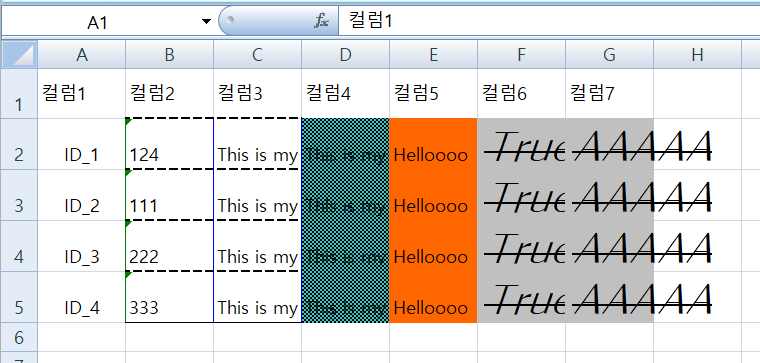
5. 사용자 맞춤형 시트 만들기
목표로 하는 엑셀을 다음 처럼 하고 싶은 것입니다.
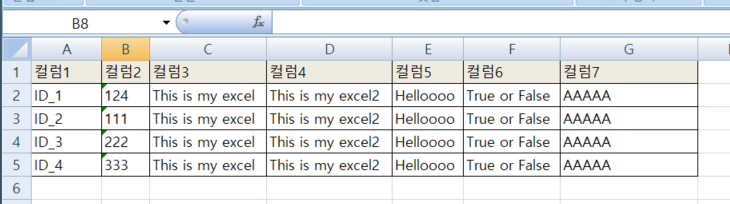
우선 헤더 셀을 만드는 메서드와 일반 셀을 만드는 메서드를 하나씩 만들었죠.
private static void createHeaderCell(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
// Create a new font and alter it.
Font font = wb.createFont();
//font.setFontHeightInPoints((short)24);
font.setFontName("Gulim");
font.setItalic(true);
font.setStrikeout(true);
font.setBold(true);
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Style the cell with borders all around.
CellStyle style = wb.createCellStyle();
style.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style.setBorderBottom(BorderStyle.THIN);
style.setBottomBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderLeft(BorderStyle.THIN);
style.setLeftBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderRight(BorderStyle.THIN);
style.setRightBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderTop(BorderStyle.THIN);
style.setTopBorderColor(IndexedColors.BLACK.getIndex());
style.setAlignment(halign);
style.setVerticalAlignment(valign);
style.setFont(font);
cell.setCellStyle(style);
}
private static void createCommonCell(
Workbook wb, Row row, int column,
HorizontalAlignment halign, VerticalAlignment valign,
String value)
{
// Create a new font and alter it.
Font font = wb.createFont();
//font.setFontHeightInPoints((short)24);
font.setFontName("Gulim");
font.setItalic(true);
font.setStrikeout(true);
Cell cell = row.createCell(column);
cell.setCellValue(value);
// Style the cell with borders all around.
CellStyle style = wb.createCellStyle();
//style.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
//style.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style.setBorderBottom(BorderStyle.THIN);
style.setBottomBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderLeft(BorderStyle.THIN);
style.setLeftBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderRight(BorderStyle.THIN);
style.setRightBorderColor(IndexedColors.BLACK.getIndex());
style.setBorderTop(BorderStyle.THIN);
style.setTopBorderColor(IndexedColors.BLACK.getIndex());
style.setAlignment(halign);
style.setVerticalAlignment(valign);
style.setFont(font);
cell.setCellStyle(style);
}
저의 경험상 일반적으로 이렇게 꾸미기를 많이 해 주면, 시스템 부담이나 혹은 오류의 가능성이 높아지기는 할 것 같네요. 하지만, 내부를 전혀 모르기 때문에 원하는 형식을 맞추기 위해서 노력 할 뿐~
셀 안에 텍스트가 해당 셀에서 가리지 않도록 처리 하는 방법에 대해서 찾아 보았습니다.
다음은 별거 없는 테스트 코드 입니다.
public static void exportMyDataToExcel3(List<MyExcelObj> myexcelObjList)
{
String excelFilePath = "3)myexcel_${count}.xlsx".replace("${count}",String.valueOf(myexcelObjList.size()));
Workbook workbook = null;
Sheet sheet = null;
BufferedOutputStream outputStream = null;
final String sheetName = "MyFirstSheet";
final String headerNames[][]
= new String[][]{
{"컬럼1", "컬럼2", "컬럼3", "컬럼4", "컬럼5", "컬럼6", "컬럼7"},
{"Col1", "Col2", "Col3", "Col4", "Col5", "Col6", "Col7"}
};
final int numberOfColumns = headerNames[0].length;
try
{
workbook = new XSSFWorkbook();
sheet = workbook.createSheet(sheetName);
Row headerRow = sheet.createRow(0);
//headerRow.setHeightInPoints(30);
for (int i = 0; i < numberOfColumns; i++) {
createHeaderCell(workbook, headerRow, i, HorizontalAlignment.CENTER, VerticalAlignment.TOP, headerNames[0][i]);
}
} catch (Exception e) {
System.out.println("File IO error:");
e.printStackTrace();
}
String Col1 = null;
String Col2 = null;
String Col3 = null;
String Col4 = null;
String Col5 = null;
String Col6 = null;
String Col7 = null;
MyExcelObj selfObj = null;
try
{
int rowCount = 1;
for(Iterator<MyExcelObj> checkIter = myexcelObjList.iterator(); checkIter.hasNext();)
{
selfObj = checkIter.next();
Row row = sheet.createRow(rowCount);
Col1 = selfObj.getCol1();
Col2 = selfObj.getCol2();
Col3 = selfObj.getCol3();
Col4 = selfObj.getCol4();
Col5 = selfObj.getCol5();
Col6 = selfObj.getCol6();
Col7 = selfObj.getCol7();
createCommonCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCommonCell(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCommonCell(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCommonCell(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCommonCell(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCommonCell(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCommonCell(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
sheet.autoSizeColumn(0);
sheet.autoSizeColumn(1);
sheet.autoSizeColumn(2);
sheet.autoSizeColumn(3);
sheet.autoSizeColumn(4);
sheet.autoSizeColumn(5);
sheet.autoSizeColumn(6);
rowCount++;
Col1 = null;
Col2 = null;
Col3 = null;
Col4 = null;
Col5 = null;
Col6 = null;
Col7 = null;
}
outputStream = new BufferedOutputStream(new FileOutputStream(excelFilePath));
workbook.write(outputStream);
} catch (IOException e) {
System.out.println("File IO error:");
e.printStackTrace();
}
finally
{
try {
if(workbook != null) workbook.close();
if(outputStream != null) outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
결과치는 ?
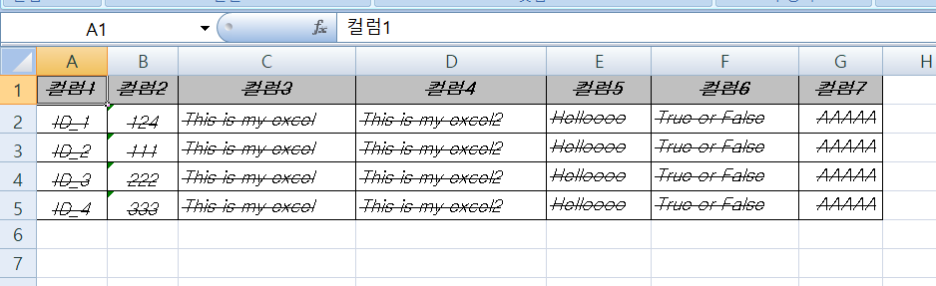
뭐 글자가 이렇게 나오긴 하지만... 뭐 여러가지 차선 책이 있기 때문에 건너뛰고 대충 아름다운 모습이네요.
6. 시트 여러개 만들기
뭐 시트를 하나씩 열어서 만드는 방법이 있겠으나, 그건 약간의 한계를 만들어 낼 수가 있으니, 시트를 여러 개 만들어서 하나의 엑셀을 만들어 보면 되겠습니다.
아까 예제에서 리스트를 네개 만들어서 한 번씩 더 집어 넣도록 하겠습니다.
myxcelList.add(obj1);
myxcelList.add(obj2);
myxcelList.add(obj3);
myxcelList.add(obj4);
myxcelList2.add(obj1);
myxcelList2.add(obj2);
myxcelList2.add(obj3);
myxcelList2.add(obj4);
myxcelList3.add(obj1);
myxcelList3.add(obj2);
myxcelList3.add(obj3);
myxcelList3.add(obj4);
myxcelList4.add(obj1);
myxcelList4.add(obj2);
myxcelList4.add(obj3);
myxcelList4.add(obj4);
그리고 이름이 필요 하니 HashMap 을 사용 할까요? 이렇게요...
String name = "myxcelList";
myxcelList.add(obj1);
myxcelList.add(obj2);
myxcelList.add(obj3);
myxcelList.add(obj4);
String name2 = "myxcelList2";
myxcelList2.add(obj1);
myxcelList2.add(obj2);
myxcelList2.add(obj3);
myxcelList2.add(obj4);
String name3 = "myxcelList3";
myxcelList3.add(obj1);
myxcelList3.add(obj2);
myxcelList3.add(obj3);
myxcelList3.add(obj4);
String name4 = "myxcelList4";
myxcelList4.add(obj1);
myxcelList4.add(obj2);
myxcelList4.add(obj3);
myxcelList4.add(obj4);
Map<String, List<TobeeExcelSheet.MyExcelObj>> MyxcelTable = new HashMap<String, List<TobeeExcelSheet.MyExcelObj>>();
MyxcelTable.put(name, myxcelList);
MyxcelTable.put(name2, myxcelList2);
MyxcelTable.put(name3, myxcelList3);
MyxcelTable.put(name4, myxcelList4);
exportMyDataTableToExcel 메서드를 하나 만들어 봅시다.
대충,
- 키 값은 시트의 이름이 되고,
- 각 value 는 리스트 형태로 기존 만들어 놓은 메서드를 약간 변경
하도록 하겠습니다
주요 흐름은
- Map 에 들어온 개수 만큼 sheet 를 생성 하고,
- 해당 sheet 에 데이터를 저장 하고 난 다음,
- 파일 스트림으로 내 보는 것
입니다.
헤더 파일의 내용은 고정 되어 있고 모든 sheet 에서 동일하다 라는 전제로 다음 코드가 구성 되었습니다.
public static void exportMyDataTableToExcel(final String[][] headerNames, Map<String, List<MyExcelObj>> myxcelTable) {
Set<String> keySet = myxcelTable.keySet();
String keyStr[] = new String[keySet.size()];
keyStr = keySet.toArray(keyStr);
Sheet sheets[] = new Sheet[keyStr.length];
Workbook workbook = null;
Sheet sheet = null;
BufferedOutputStream outputStream = null;
final int numberOfColumns = headerNames[0].length;
int totCount = 0;
workbook = new XSSFWorkbook();
for(int i = 0; i < keyStr.length; i++)
{
sheets[i] = workbook.createSheet(keyStr[i]);
}
for(int i = 0; i < sheets.length; i++)
{
sheet = sheets[i];
Row headerRow = sheet.createRow(0);
//headerRow.setHeightInPoints(30);
for (int j = 0; j < numberOfColumns; j++) {
createHeaderCell(workbook, headerRow, j, HorizontalAlignment.CENTER, VerticalAlignment.TOP, headerNames[0][j]);
}
String Col1 = null;
String Col2 = null;
String Col3 = null;
String Col4 = null;
String Col5 = null;
String Col6 = null;
String Col7 = null;
MyExcelObj selfObj = null;
List<MyExcelObj> myexcelObjList = myxcelTable.get(keyStr[i]);
int rowCount = 1;
for(Iterator<MyExcelObj> checkIter = myexcelObjList.iterator(); checkIter.hasNext();)
{
selfObj = checkIter.next();
Row row = sheet.createRow(rowCount);
Col1 = selfObj.getCol1();
Col2 = selfObj.getCol2();
Col3 = selfObj.getCol3();
Col4 = selfObj.getCol4();
Col5 = selfObj.getCol5();
Col6 = selfObj.getCol6();
Col7 = selfObj.getCol7();
createCommonCell(workbook, row, 0, HorizontalAlignment.CENTER, VerticalAlignment.BOTTOM, Col1);
createCommonCell(workbook, row, 1, HorizontalAlignment.CENTER_SELECTION, VerticalAlignment.BOTTOM, Col2);
createCommonCell(workbook, row, 2, HorizontalAlignment.FILL, VerticalAlignment.CENTER, Col3);
createCommonCell(workbook, row, 3, HorizontalAlignment.GENERAL, VerticalAlignment.CENTER, Col4);
createCommonCell(workbook, row, 4, HorizontalAlignment.JUSTIFY, VerticalAlignment.JUSTIFY, Col5);
createCommonCell(workbook, row, 5, HorizontalAlignment.LEFT, VerticalAlignment.TOP, Col6);
createCommonCell(workbook, row, 6, HorizontalAlignment.RIGHT, VerticalAlignment.TOP, Col7);
sheet.autoSizeColumn(0);
sheet.autoSizeColumn(1);
sheet.autoSizeColumn(2);
sheet.autoSizeColumn(3);
sheet.autoSizeColumn(4);
sheet.autoSizeColumn(5);
sheet.autoSizeColumn(6);
rowCount++;
totCount++;
Col1 = null;
Col2 = null;
Col3 = null;
Col4 = null;
Col5 = null;
Col6 = null;
Col7 = null;
}
}
String excelFilePath = "4)myexcel_${count}.xlsx".replace("${count}",String.valueOf(totCount));
try {
outputStream = new BufferedOutputStream(new FileOutputStream(excelFilePath));
workbook.write(outputStream);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
finally
{
try {
if(workbook != null) workbook.close();
if(outputStream != null) outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
결과 치는 탭에 얌전히 들어가 있는 시트들을 볼 수 있다는 것입니다...
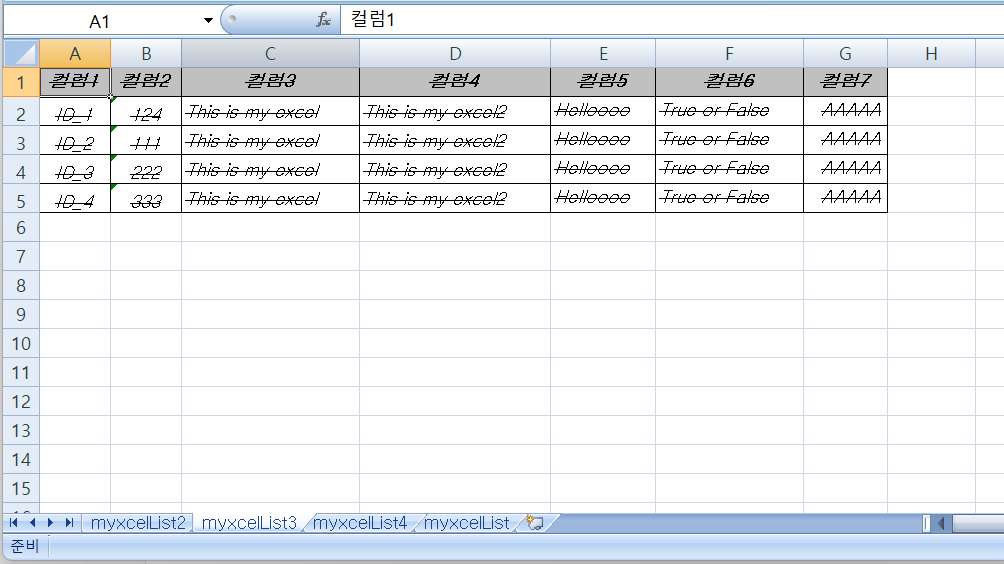
설명할 내용은 그리 많지 않아서, 생각이 나면 다시 글을 업데이트 하는 것으로 하는 것이 좋을 듯 하네요.
오늘은 아파치 POI 모듈을 사용해서, 엑셀을 만들어 보았습니다.
이상.
'프로그래밍' 카테고리의 다른 글
Nodejs 로 Http GET 메시지 테스트 하기 (1) | 2022.09.10 |
---|---|
아파치 POI로 하는 엑셀 프로그래밍-2 (0) | 2022.09.07 |
Guice - Google (2) | 2022.08.30 |
DisconnectedContext 오류 (0) | 2022.04.28 |
[자바]특정 이미지에 투명도 입히기 (0) | 2022.04.11 |