Android Notification은 애플리케이션이 실행되고 있지 않더라도 애플리케이션에서 발생한 작업에 대한 짧고 시기적절한 정보를 제공합니다. 알림은 아이콘, 제목 및 일정량의 콘텐츠 텍스트를 표출 합니다.
Android Notification 속성 설정
Android 알림의 속성은 NotificationCompat.Builder 개체를 사용하여 설정됩니다. 알림 속성 중 일부는 아래와 같습니다:
- setSmallIcon(): 알림 아이콘을 설정합니다.
- setContentTitle(): 알림 제목을 설정하는데 사용됩니다.
- setContentText(): 텍스트 메시지를 설정하는데 사용됩니다.
- setAutoCancel(): 알림의 취소 가능 속성을 설정합니다.
- setPriority(): 알림 우선순위를 설정합니다.
Android Notification 예제
이 예에서는 우리는 클릭하면, 다른 액티비티를 시작하는 알림 메시지를 생성 할 것입니다.
activity_main.xml
activity_main.xml 파일에 다음 코드를 추가합니다.
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="example.javatpoint.com.androidnotification.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="ANDROID NOTIFICATION"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.091"
android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/button"
android:layout_marginBottom="112dp"
android:layout_marginEnd="8dp"
android:layout_marginStart="8dp"
android:text="Notify"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</android.support.constraint.ConstraintLayout>
activity_notification_view.xml이라는 액티비티를 만들고 다음 코드를 추가합니다. 이 액티비티는 알림을 클릭하면 시작됩니다. TextView는 알림 메시지를 표시하는 데 사용됩니다.
activity_notification_view.xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="example.javatpoint.com.androidnotification.NotificationView">
<TextView
android:id="@+id/textView2"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="your detail of notification..."
android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="8dp"
android:layout_marginEnd="8dp"
android:layout_marginStart="8dp"
android:layout_marginTop="8dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.096"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView2"
app:layout_constraintVertical_bias="0.206"
android:textAppearance="@style/Base.TextAppearance.AppCompat.Medium"/>
</android.support.constraint.ConstraintLayout>
MainActivity.java
MainActivity.java 클래스에 다음 코드를 추가합니다. 이 클래스에서 버튼을 클릭하면 알림 속성을 설정하기 위해 NotificationCompat.Builder 객체를 구현하는 addNotification() 메서드가 호출됩니다. NotificationManager.notify() 메서드는 알림을 표시하는 데 사용됩니다. Intent 클래스는 알림을 탭할 때 다른 액티비티를(NotificationView.java) 호출하는 데 사용됩니다.
package example.javatpoint.com.androidnotification;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.content.Context;
import android.content.Intent;
import android.support.v4.app.NotificationCompat;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
Button button;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
addNotification();
}
});
}
private void addNotification() {
NotificationCompat.Builder builder =
new NotificationCompat.Builder(this)
.setSmallIcon(R.drawable.messageicon) //set icon for notification
.setContentTitle("Notifications Example") //set title of notification
.setContentText("This is a notification message")//this is notification message
.setAutoCancel(true) // makes auto cancel of notification
.setPriority(NotificationCompat.PRIORITY_DEFAULT); //set priority of notification
Intent notificationIntent = new Intent(this, NotificationView.class);
notificationIntent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP);
//notification message will get at NotificationView
notificationIntent.putExtra("message", "This is a notification message");
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent,
PendingIntent.FLAG_UPDATE_CURRENT);
builder.setContentIntent(pendingIntent);
// Add as notification
NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
manager.notify(0, builder.build());
}
}
NotificationView.java
NotificationView.java 클래스는 알림 메시지를 수신하고 TextView에 표시됩니다. 이 클래스는 알림을 탭 할 때, 호출됩니다.
package example.javatpoint.com.androidnotification;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.TextView;
import android.widget.Toast;
public class NotificationView extends AppCompatActivity {
TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_notification_view);
textView = findViewById(R.id.textView);
//getting the notification message
String message=getIntent().getStringExtra("message");
textView.setText(message);
}
}
strings.xml
<resources>
<string name="app_name">AndroidNotification</string>
<string name="notification_activity">NotificationView</string>
</resources>
AndroidManifest.xml
AndroidManifest.xml 파일에 다음 코드를 추가합니다.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="example.javatpoint.com.androidnotification">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<activity android:name=".NotificationView"
android:label="@string/notification_activity"
android:parentActivityName=".MainActivity">
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value=".MainActivity"/>
</activity>
</application>
</manifest>
출력 결과:
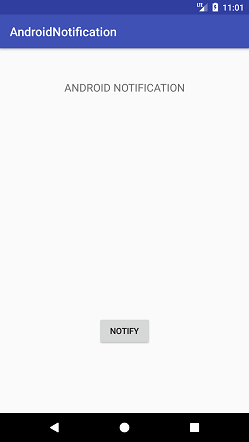
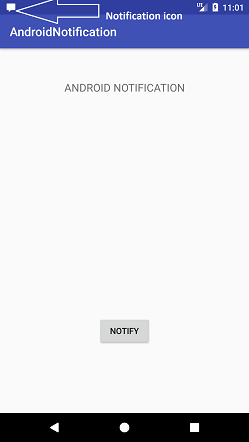
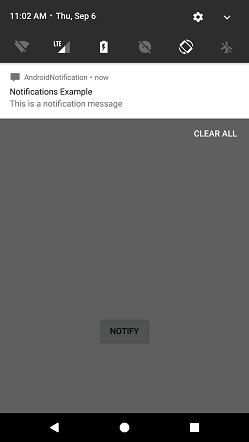
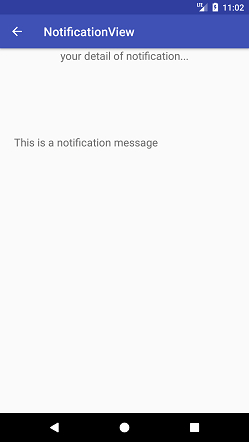
이상.
'모바일프로그래밍 > 안드로이드' 카테고리의 다른 글
안드로이드 APK 분석 참고 문서 (0) | 2023.01.04 |
---|---|
안드로이드 이해하기 - 첫 걸음 떼기 (0) | 2023.01.02 |
LogCat을 이용한 안드로이드 디버깅 (0) | 2022.11.11 |
안드로이드 기기 디버깅을 위한 크롬브라우저 설정 (0) | 2022.09.18 |
안드로이드 APK 분석 참고 문서 (0) | 2022.09.15 |