728x90
저번 글에서 만들어 낸 TSP 순서를 나타내는 결과는 시작적인 정보가 조금 약하다는 생각이 듭니다.
https://tobee.tistory.com/entry/OSRM-vroom%EA%B3%BC-OSRM%EC%9D%84-%EC%9D%B4%EC%9A%A9%ED%95%9C-TSP
TSP 결과 시각정보 강화해 보기
그래서 이 알고리즘을 만들어 준 순번을 표출 할 수 있도록 코드를 다음과 같이 변경해 보겠습니다.
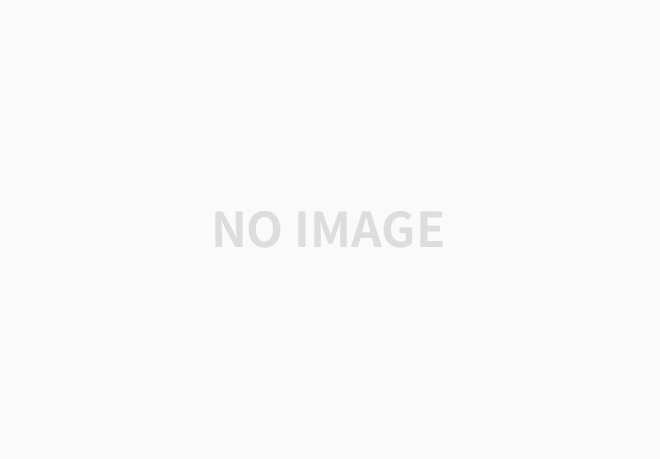
코드는 다음과 같습니다. - tsp_viewer_with_id.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Leaflet Map with Job Routes and Job IDs</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" />
<style>
#map {
height: 100vh;
}
/* Custom style for the job ID label */
.id-label {
background-color: rgba(255, 255, 255, 0.8); /* White background with some transparency */
border: 2px solid red; /* Red border */
border-radius: 5px; /* Rounded corners */
padding: 5px 10px; /* Padding to make the label more readable */
font-size: 16px; /* Font size */
color: red; /* Red text color */
font-weight: bold; /* Bold text */
}
</style>
</head>
<body>
<div id="map"></div>
<script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js"></script>
<script>
// Initialize the map
const map = L.map('map').setView([37.497942, 127.027621], 13); // Gangnam, Seoul
// OpenStreetMap tile layer
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
maxZoom: 19,
}).addTo(map);
// Load the output.json file and process it
fetch('output.json')
.then(response => response.json())
.then(data => {
// Array to hold the job locations
const jobLocations = [];
// Iterate over each step in the routes
data.routes.forEach(route => {
route.steps.forEach((step, index) => {
if (step.type === "job" && step.id) { // Only process "job" steps
// Create a label with only the job ID (number only)
const label = L.divIcon({
className: 'id-label',
html: `${step.id}`, // Display the job id as a number only
});
// Create a marker at the job's location with the label
L.marker([step.location[1], step.location[0]], { icon: label }).addTo(map);
// Save the job location (latitude, longitude)
jobLocations.push([step.location[1], step.location[0]]);
}
});
});
// Draw blue connecting lines between consecutive job locations
for (let i = 0; i < jobLocations.length - 1; i++) {
// Create a polyline (blue connecting line) between consecutive job locations
L.polyline([jobLocations[i], jobLocations[i + 1]], {
color: 'blue',
weight: 3,
opacity: 0.7
}).addTo(map);
}
// Optionally, set the map bounds to fit all the job locations
const bounds = L.latLngBounds(jobLocations);
map.fitBounds(bounds);
})
.catch(err => console.error('Error loading output.json:', err));
</script>
</body>
</html>
TSP 결과 경로정보 시각정보 강화해 보기
그런 다음, 이 결과에 따라 정리 된 TSP 경로도 위와 같이 시각화 해보기로 했습니다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Leaflet Map with Job Routes and Job IDs</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet@1.9.4/dist/leaflet.css" />
<style>
#map {
height: 100vh;
}
/* Custom style for the job ID label */
.id-label {
background-color: rgba(255, 255, 255, 0.8); /* White background with some transparency */
border: 2px solid red; /* Red border */
border-radius: 5px; /* Rounded corners */
padding: 5px 10px; /* Padding to make the label more readable */
font-size: 16px; /* Font size */
color: red; /* Red text color */
font-weight: bold; /* Bold text */
}
</style>
</head>
<body>
<div id="map"></div>
<script src="https://unpkg.com/leaflet@1.9.4/dist/leaflet.js"></script>
<script>
// Initialize the map
const map = L.map('map').setView([37.497942, 127.027621], 13); // Gangnam, Seoul
// OpenStreetMap tile layer
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
maxZoom: 19,
}).addTo(map);
// Load the output.json file and process it
fetch('output.json')
.then(response => response.json())
.then(data => {
// Array to hold the job locations
const jobLocations = [];
// Iterate over each step in the routes
data.routes.forEach(route => {
route.steps.forEach((step, index) => {
if (step.type === "job" && step.id) { // Only process "job" steps
// Create a label with only the job ID (number only)
const label = L.divIcon({
className: 'id-label',
html: `${step.id}`, // Display the job id as a number only
});
// Create a marker at the job's location with the label
L.marker([step.location[1], step.location[0]], { icon: label }).addTo(map);
// Save the job location (latitude, longitude)
jobLocations.push([step.location[1], step.location[0]]);
}
});
});
// Draw blue connecting lines between consecutive job locations
for (let i = 0; i < jobLocations.length - 1; i++) {
// Create a polyline (blue connecting line) between consecutive job locations
L.polyline([jobLocations[i], jobLocations[i + 1]], {
color: 'blue',
weight: 3,
opacity: 0.7
}).addTo(map);
}
// Optionally, set the map bounds to fit all the job locations
const bounds = L.latLngBounds(jobLocations);
map.fitBounds(bounds);
})
.catch(err => console.error('Error loading output.json:', err));
</script>
</body>
</html>
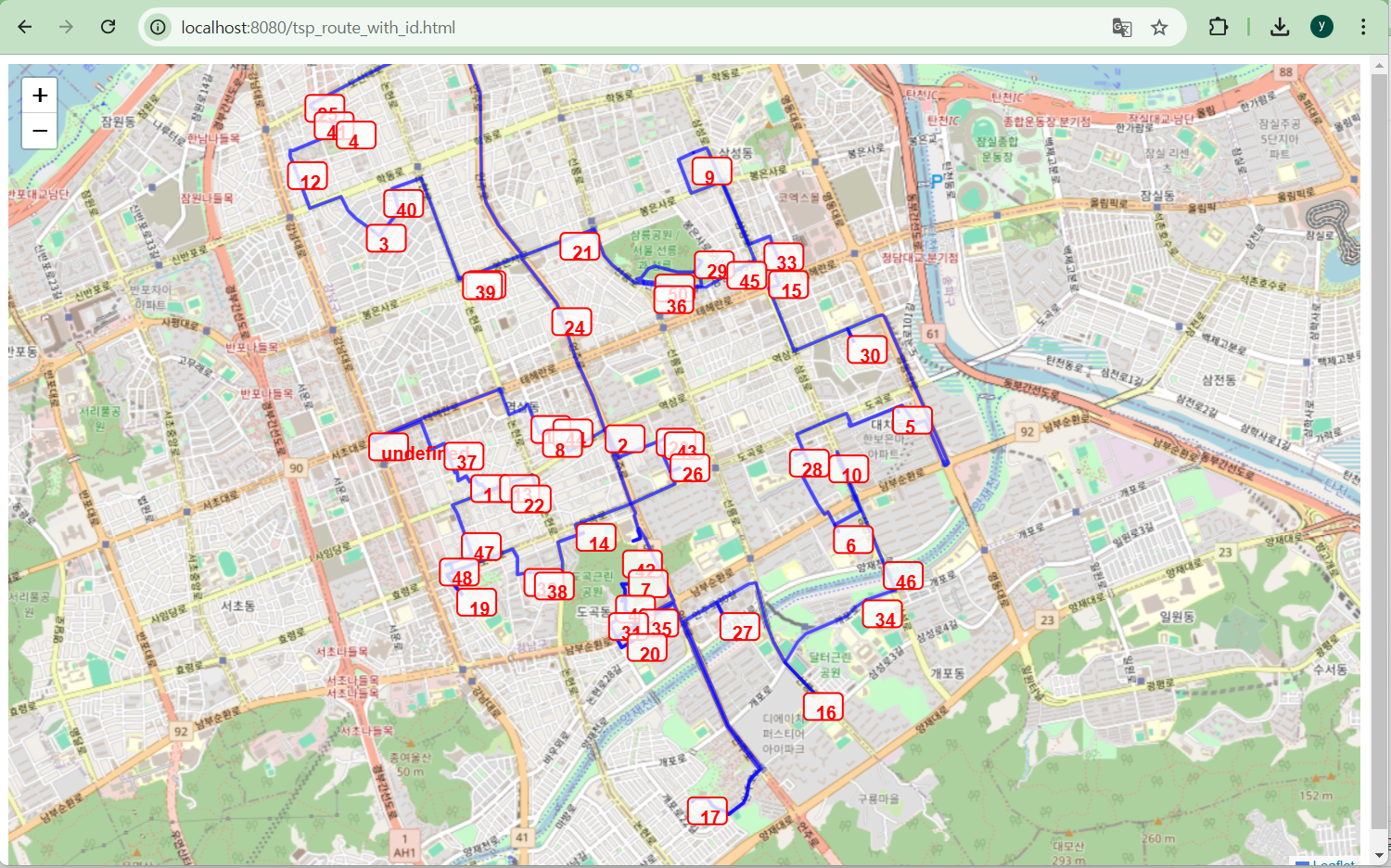
이로써 내가 생각하는 TSP 알고리즘을 위한 시스템 구현이 끝난 것 같네요...
그럼 이만...
728x90
'프로그래밍 > GIS' 카테고리의 다른 글
[OSRM] Chat GPT와 함께하는 TSP - 지도 표시 (1) | 2024.12.09 |
---|---|
[OSRM] Chat GPT와 함께하는 TSP - 마무리 (1) | 2024.12.06 |
[OSRM] Chat GPT와 함께하는 TSP - 4 (0) | 2024.12.04 |
[OSRM] Chat GPT와 함께하는 TSP - 3 (1) | 2024.12.03 |
vroom - Vehicle Routing Open-source Optimization Machine (0) | 2024.11.30 |