https://www.codeproject.com/Tips/5345379/Scientific-Software-in-Your-Hand
Scientific Software in Your Hand
Science and programming on working examples
www.codeproject.com
사이언스 프로그래밍 실행 예제
일반적인 프로그램 솔루션을 위한 효과적인 알고리즘을 제시합니다.
소개
이 짧은 팁에서 우리는 소프트웨어의 프로그래밍 및 개발에서 사이언스와 연구의 중요성에 대해서 소개 해 볼까 합니다.
소프트웨어 목록은 다음과 같습니다.
- EasyBlockchain - 디지털 지갑
- Fiesta - 바이러스 백신 프로그램
- ProjectEnv - 오픈 스토리지가 있는 모델 최적화 소프트웨어
- TestKit - 자체 평가 프로그램
- Zines - 대략적인 텍스트 검색 프로그램
위 목록에 있는 4개의 프로그램은 C#으로 개발되었으며 Zines는 Java 프로젝트입니다.
배경
문자열 검색이나 해시 계산과 같이 이전에 설명한 많은 알고리즘이 있지만 이 팁에서 제시한 알고리즘보다 효율성이 떨어집니다.
코드 사용
먼저 디지털 지갑에서 블록체인 작업을 살펴봅시다:
public Blockchain Operation(float f) {
int fi = (int) (f * 100.0f);
string value = "";
int c = fi;
for (int i = 0; i < this.Value.Length; ++i) {
int k = valueOf(this.Value[i]) + c;
c = 0;
while (k < 0) {
k += 16;
--c;
}
while (k >= 16) {
k -= 16;
++c;
}
value += "0123456789abcdef"[k];
}
Blockchain result = new Blockchain(value);
return result;
}
위 코드에서 볼 수 있듯이 선형적인 성능을 보이며 배정밀도로 값을 처리합니다.
다음 코드는 디지털 저장소의 값을 얻기 위해 두 해시 값의 빼기를 처리합니다:
public bool Less(Blockchain bc) {
for (int i = this.Value.Length - 1; i >= 0; --i) {
if (valueOf(this.Value[i]) != valueOf(bc.Value[i])) {
return valueOf(this.Value[i]) < valueOf(bc.Value[i]);
}
}
return false;
}
public float Subtract(Blockchain bc) {
float result = 0;
float p = 1.0f;
int c = 0;
if (this.Less(bc)) {
return -bc.Subtract(this);
}
for (int i = 0; i < this.Value.Length; ++i) {
int a = valueOf(this.Value[i]);
int b = valueOf(bc.Value[i]);
int d = a - b + c;
c = 0;
while (d < 0) {
d += 16;
--c;
}
while (d >= 16) {
d -= 16;
++c;
}
result += p * d;
p *= 16.0f;
}
return result / 100.0f;
}
여기서 Blockchain 클래스의 값은 문자열입니다.
Fiesta 바이러스 백신 소프트웨어는 또한 선형 해시를 계산하므로 이 절차의 가장 최적의 방법을 활용합니다:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace Fiesta
{
public class Hash
{
public const int HASH_SIZE = 128;
public static byte[] GetHash(Stream stream)
{
int current = 0, b = -1;
byte[] result = new byte[HASH_SIZE];
for (int i = 0; i < result.Length; ++i)
{
result[i] = 0;
}
while ((b = stream.ReadByte()) != -1)
{
result[current] = (byte) ((int) result[current] ^ b);
current = (current + 1) % HASH_SIZE;
}
return result;
}
}
}
이 해시는 또 다른 동일한 해시를 검색하기 위해 #ries 또는 Aho-Corasick 트리 내에서 사용됩니다.
ProjectEnv 응용 프로그램은 변수 수의 전체 분산을 최적화하는 것을 목표로 하며 동적 프로그래밍(DP) 접근 방식을 사용하여 해결됩니다:
public void Optimize(int m)
{
int n = 1;
for (int i = 0; i < this.Factors.Count; ++i)
{
Factor f = (Factor)this.Factors[i];
n += (int)Math.Round(f.Dispersion * 100.0);
}
int[,] opt = new int[n, m + 1];
int[,] next_n = new int[n, m + 1];
int[,] next_m = new int[n, m + 1];
for (int i = 0; i < n; ++i)
{
for (int j = 0; j <= m; ++j)
{
opt[i, j] = -1;
next_n[i, j] = -1;
next_m[i, j] = -1;
}
}
opt[0, 0] = 0;
for (int i = 0; i < this.Factors.Count; ++i)
{
Factor f = (Factor)this.Factors[i];
int d = (int)Math.Round(f.Dispersion * 100.0);
f.IsOptimal = false;
for (int k = m; k >= 1; --k)
{
for (int j = n - 1; j >= 0; --j)
{
if (j >= d && opt[j - d, k - 1] != -1 && opt[j, k] == -1)
{
opt[j, k] = opt[j - d, k - 1] + d;
next_n[j, k] = j - d;
next_m[j, k] = i;
}
}
}
}
int found = -1;
for (int i = 0; i < n; ++i)
{
if (opt[i, m] >= 0)
{
found = i;
break;
}
}
if (found >= 0)
{
for (int i = m; i > 0; --i)
{
Factor f = (Factor)this.Factors[next_m[found, i]];
f.IsOptimal = true;
found = next_n[found, i];
}
}
}
TestKit 프로그램은 HTML(HyperText Markup Language)에서 추가로 사용할 객체 모델을 얻기 위해 구문 분석 기술을 사용합니다:
using System;
using System.Collections;
using System.Collections.Generic;
namespace TestKit
{
/// <summary>
/// Question class.
/// </summary>
public class Question
{
public string text;
public ArrayList answers = new ArrayList();
public Question(Stream stream)
{
this.text = "";
while (!stream.atEnd() && stream.current != ':') {
this.text += (char) stream.current;
stream.Read();
}
stream.Read();
this.text = this.text.Trim();
while (!stream.atEnd()) {
Answer answer = new Answer(stream);
this.answers.Add(answer);
if (answer.isFinal) break;
}
}
}
}
Answer 클래스는 구문 분석 기술 내에서 위와 동일하게 정의됩니다:
using System;
namespace TestKit
{
/// <summary>
/// Answer class.
/// </summary>
public class Answer
{
public string text;
public bool isFinal = false;
public bool isCorrect = false;
public static void parseBlanks(Stream stream) {
while (" \r\n\t\v".IndexOf((char) stream.current) >= 0 && !stream.atEnd()) {
stream.Read();
}
}
public Answer(Stream stream)
{
parseBlanks(stream);
if (stream.current == '+') {
this.isCorrect = true;
stream.Read();
}
this.text = "";
while (!stream.atEnd() && stream.current != ';') {
if (stream.current == '.') {
this.isFinal = true;
break;
}
this.text += (char) stream.current;
stream.Read();
}
stream.Read();
this.text = this.text.Trim();
}
}
}
Zines Java 프로그램은 슬라이딩 윈도우와 함께 근사 알고리즘을 사용하고 다음과 같이 정의된 Result 클래스를 활용합니다:
package com.zines;
public class Result {
public String path = null;
public int length = -1;
public int offset = -1;
public String query = null;
public float ratio = 0.0f;
public String text = null;
public Result() {
}
public static void printlnHeader() {
System.out.println("\"FILE\",\"OFFSET\",\"LENGTH\",\"RATIO\"");
}
public void println() {
System.out.println("\""
+ this.path + "\","
+ this.offset
+ ","
+ this.length + ","
+ this.ratio + "");
}
}
관심 주제들
따라서 우리는 불필요한 과도한 코드를 작성하지 않고 최적화 및 쉬운 해결 방법과 같은 문제를 해결하기 위해 사이언스와 프로그래밍이 어떻게 함께 운용 될 수 있는 지에 대해서 확인 해 보았습니다.
하지만 위의 모든 내용에서 과학적인 접근 방식이 없는 경우에도 최신 알고리즘이 여전히 적용 가능하다는 결론이 나옵니다.
History
- 27th October, 2022 - Initial release
- 2nd November, 2022 - Removed links
- 3rd November, 2022 - Links deleted
- 4th November, 2022 - License changed
License
This article, along with any associated source code and files, is licensed under The Code Project Open License (CPOL)
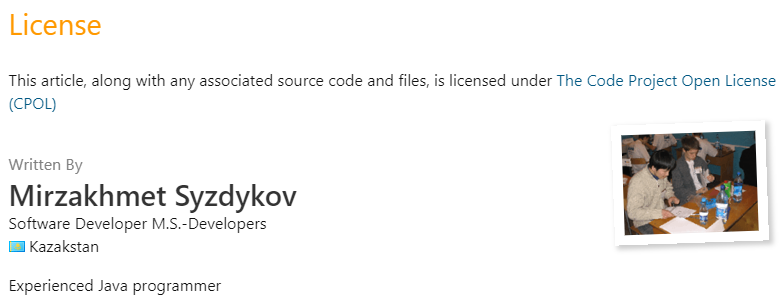
이상.
'프로그래밍' 카테고리의 다른 글
빠른 2:1 이미지 축소(축소) (0) | 2022.12.14 |
---|---|
C++ 심층 네트워크 프로그래밍 (1) | 2022.12.13 |
웹 요청 순서 시각화 (0) | 2022.12.11 |
뮤텍스, 세마포어 그리고 끔찍한 헤어스타일 사이 (0) | 2022.12.10 |
데이터 사이언스을 위한 C/C++ (1) | 2022.12.08 |